Have you been struggling with some NPM library providing async
API? It is surprisingly easy to set up a playground capable of Javascript async
, await
. Let's get to it!
Solution
It is quite a straight forward process with one key aspect. You want to initialize new NPM package and prepare script that is capable to run asynchronous API and wait for the result.
I suppose you already have Node.js installed since you want to test out some javascript locally.
First, you need to create empty directory and initialize the NPM package inside.
mkdir playground
cd playground
npm init -y
This will create package.json
file with this content.
{
"name": "playground",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Second step is to create index.js
file with self-invocation function plus mark this function as async! This will do the trick and you could use await
wherever you want.
const doWhatever = async () => {
// await whatever
};
// Self-invocation async function
(async () => {
await doWhatever();
})().catch((err) => {
console.error(err);
throw err;
});
Last step is to add a NPM script that will run index.js
file to test it out. Just define start
script that will run node index.js
command.
{
"name": "playground",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start": "node index.js" // THAT LINE
},
"keywords": [],
"author": "",
"license": "ISC"
}
And just run the start
script via npm CLI
.
npm start
Real example
My exact scenario is to test out the Kontent Delivery SDK for Javascript for loading one content piece asynchronously using await
so the result look like that.
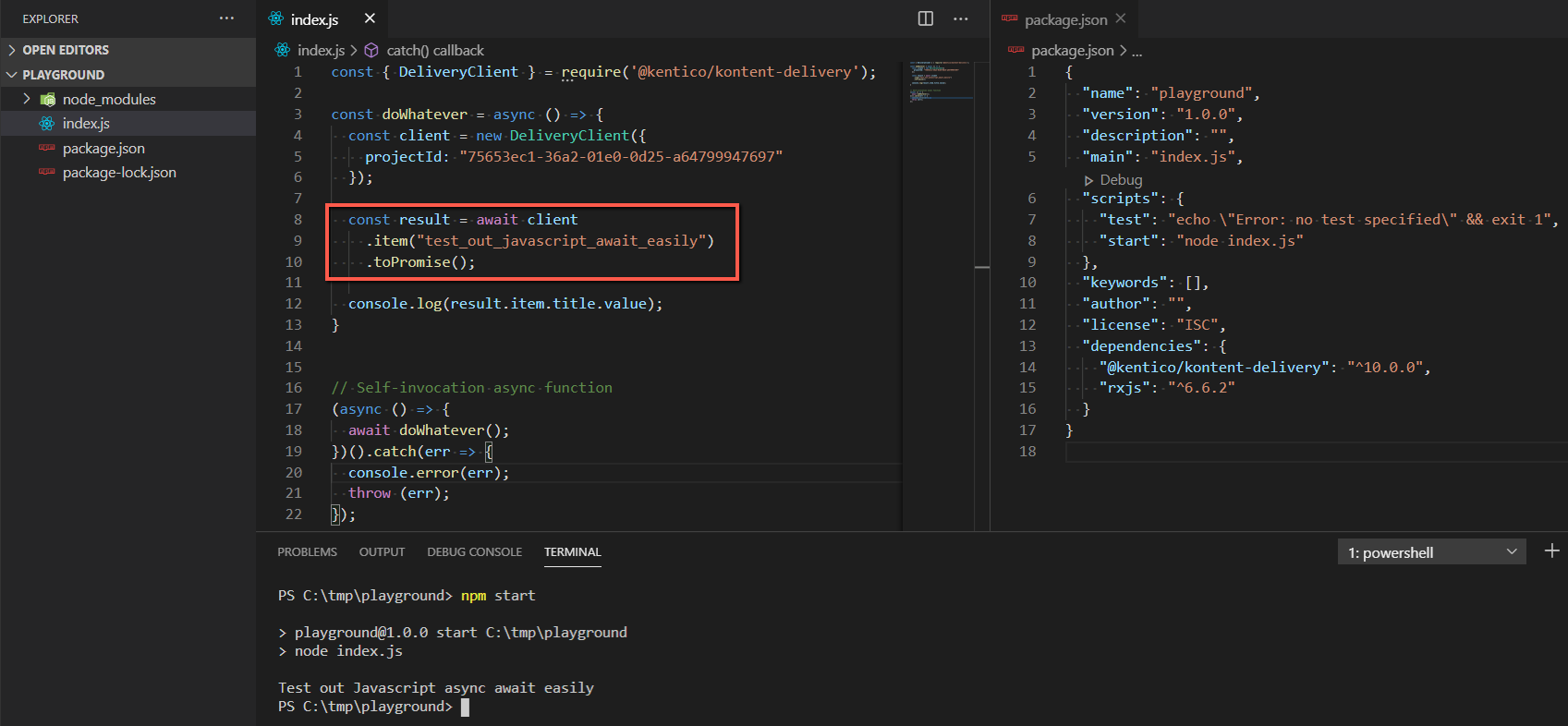
Install required packages rxjs
and @kentico/kontent-delivery
npm i rxjs --save
npm i @kentico/kontent-delivery --save
This will define these two dependencies to package.json
file and install them to node_modules
folder.
{
"name": "playground",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start": "node index.js"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": { // THESE DEPENDENCIES
"@kentico/kontent-delivery": "^10.0.0",
"rxjs": "^6.6.2"
}
}
And to test out something real, let's adjust the index.js
file to load content item of this gotcha.
const { DeliveryClient } = require('@kentico/kontent-delivery');
const doWhatever = async () => {
const client = new DeliveryClient({
projectId: "75653ec1-36a2-01e0-0d25-a64799947697",
});
const result = await client.item("test_out_javascript_await_easily")
.toPromise(); // THERE YOU GO!
console.log(result.item.title.value);
}
// Self-invocation async function
(async () => {
await doWhatever();
})().catch(err => {
console.error(err);
throw (err);
});
So by running npm start
you will get something like that!
PS C:\tmp\playground> npm start
> playground@1.0.0 start C:\tmp\playground
> node index.js
Test out Javascript async await easily
And Voila - the title logged to console! That is why we program 🤓